Use AI to Write Your Git Commit Messages
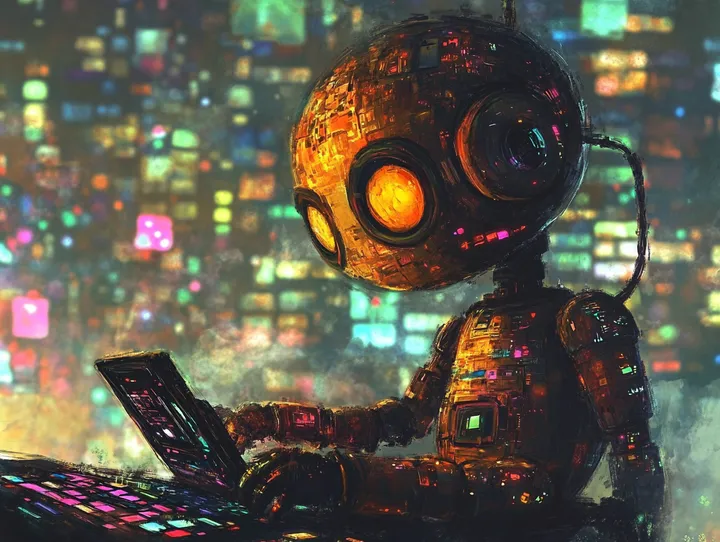
How much would you pay a person to write better git commit messages than you are willing to write?
That is a question I asked myself this morning as I was creating a series of git commits for a new feature. Sure, writing a message describing what you did when you commit a change is not terribly difficult. But, as laziness is my superpower, I wanted to see if I could get a computer to do it for me.
Here is the script I came up with:
#!/bin/bash
# source .env to get the OPENAI_API_KEY
source "$(dirname "$0")/.env"
# 📝 Get only the diff of what has already been staged
git_diff_output=$(git diff --cached)
# 🛑 Check if there are any staged changes to commit
if [ -z "$git_diff_output" ]; then
echo "⚠️ No staged changes detected. Aborting."
exit 1
fi
# 🗜️ Limit the number of lines sent to AI to avoid overwhelming it
git_diff_output_limited=$(echo "$git_diff_output" | head -n 100)
# 📦 Prepare the AI prompt for the chat model
messages=$(jq -n --arg diff "$git_diff_output_limited" '[
{"role": "system", "content": "You are an AI assistant that helps generate git commit messages based on code changes."},
{"role": "user", "content": ("Suggest an informative commit message by summarizing code changes from the shared command output. The commit message should follow the conventional commit format and provide meaningful context for future readers.\n\nChanges:\n" + $diff)}
]')
# 🚀 Send the request to OpenAI API using the correct chat endpoint
response=$(curl -s -X POST https://api.openai.com/v1/chat/completions \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $OPENAI_API_KEY" \
-d "$(jq -n \
--argjson messages "$messages" \
'{
model: "gpt-4",
messages: $messages,
temperature: 0.5,
max_tokens: 100
}'
)")
# 🔄 Extract the AI-generated commit message
commit_message=$(echo "$response" | jq -r '.choices[0].message.content' | sed 's/^ *//g')
# 🛑 Check if we got a valid commit message from the AI
if [ -z "$commit_message" ] || [[ "$commit_message" == "null" ]]; then
echo "🚫 Failed to generate a commit message from OpenAI."
echo "⚠️ API Response: $response"
exit 1
fi
# 📋 Show the suggested commit message and ask for confirmation
echo "🤖 Suggested commit message:"
echo "$commit_message"
read -p "Do you want to use this message? (y/n) " choice
if [[ "$choice" != "y" ]]; then
echo "🛑 Commit aborted by the user."
exit 1
fi
# 🛑 Option to dry run
if [[ $1 == "--dry-run" ]]; then
echo "✅ Dry run: Commit message generated, but no commit was made."
exit 0
fi
# 🔐 Commit only staged changes with the AI-generated message
if ! git commit -m "$commit_message"; then
echo "❌ Commit failed. Aborting."
exit 1
fi
# 🎉 Success message
echo "✅ Committed with message: $commit_message"
In a nutshell, this script does the following:
- Gets the diff of staged changes
- Sends the diff to OpenAI's GPT-4 model
- Receives a suggested commit message
- Asks for user confirmation
- Commits the changes with the AI-generated message
To use this script, you'll need to:
- Save it as a file (e.g.,
gitcommit
) - Make it executable (
chmod +x gitcommit
) - Put the script in an executable directory (e.g.,
/usr/local/bin
) - Create a
.env
file in the same directory with your OpenAI API key:
OPENAI_API_KEY=your_api_key_here
Run the script after staging your changes: gitcommit
This approach leverages AI to generate more descriptive and consistent commit messages, potentially improving your project's documentation and making it easier for team members to understand changes.
Some benefits of using AI for commit messages include:
- Consistency in message format
- More detailed descriptions of changes
- Time-saving for developers
- Potential for better code review processes
While this method can be helpful, it's important to review the generated messages to ensure they accurately represent your changes. You may also want to customize the prompt or fine-tune the AI model to better suit your project's specific needs.
Also, I have no idea how much this is going to cost me. I assume less than a dollar per month. That is why I asked my initial question of how much I would pay someone to write better git commit messages than I am willing to write.
By incorporating AI into your git workflow, you're not only saving time but also potentially improving the overall quality of your project's version history.
Give it a try and see how it enhances your development process!